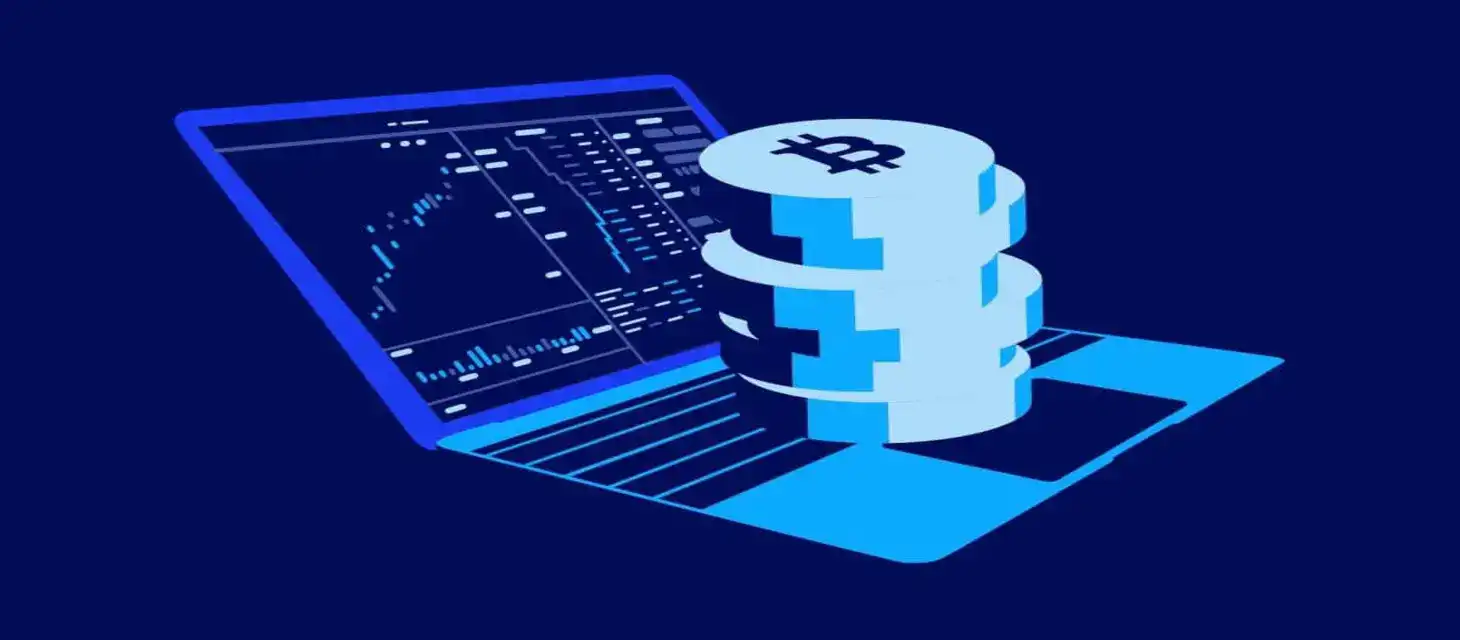
How to Build a Crypto Price Alert Telegram Bot in Golang
Table of Contents
Ever wondered how to create your very own cryptocurrency price alert Telegram bot using Golang? If so, we'll guide you through the process of building a bot that keeps you updated on the latest crypto prices, all while exploring the powerful combination of Telegram and Golang. Let's get on this coding adventure together!
So, cryptocurrency is a digital currency, which is another form of payment created using encryption algorithms used for trading, buying, and selling goods and other tokens, sometimes through crypto trading bots. However, one of the major uses of crypto through telegram bot Golang tutorial is to give awareness about the community of the crypto market crashes or cool offs. Likewise, telegram is a communication medium and one of its uses is to build bots that interact with users, groups, and channels. Here, we will learn to build a cryptocurrency alert telegram price bot using Golang.
Create a bot on Telegram
To create a bot on Telegram, we have to use BotFather which is the one of bot to rule all the bots. That is used to create new bot accounts and manage your existing bots. Now Open your telegram account and go to https://t.me/BotFather. Use the Forward slash / symbol that will give you the list of telegram bot commands.
Enter the /newbot command to create a new bot. It will ask you the name of your bot. Enter the username of the bot according to the requirements of BotFather
After that BotFather will give you the token to access the HTTP telegram bot API that we will use in our Golang program. Keep your token secure and store it safely, it can be used by anyone to control your bot.
Create a Telegram Bot Channel
We need an alert channel to send crypto price alerts by our telegram bot Golang where users get the alerts. To create a bot channel telegram, Open the Telegram app and click on the top right of the Chats bar on the Create a button that shows a popup to create a new channel.
Add Bot to Telegram Channel
Now add the created bot to the telegram channel. Open the Telegram Channel, go to channel info, click on the subscriber's button, and add the bot as a subscriber to the channel.
Now we implement bot logic in Golang that sends the crypto alert in the channel.
Install Golang
To use Golang, first install the Golang version must be 1.19 or higher. Go to the installation link: https://go.dev/doc/install. Select the OS of your local machine and download the top Golang framework and install it on your machine.
Verify the Go Version
To verify the Go installation version, enter the following command on the terminal.
go version
Create a Project
Create the Golang project to build the bot alert functionality. Now create a new folder with the name telegram-bot-golang. Open the folder in VS Code IDE and create new files main.go and prices.go in it.
Run the following commands:
go init telegram-bot-golang
go mod tidy
Paste the following code in the main.go file
package main
import (
"fmt"
"log"
"os"
"time"
tgbotapi "github.com/go-telegram-bot-api/telegram-bot-api/v5"
"github.com/joho/godotenv"
)
func main() {
err := godotenv.Load(".env")
if err != nil {
log.Fatalf("Error loading .env file")
}
bot, err := tgbotapi.NewBotAPI(os.Getenv("BOT_TOKEN"))
if err != nil {
log.Panic(err)
}
bot.Debug = true
log.Printf("Authorized on account %s", bot.Self.UserName)
sendTopCoinsMessage(bot)
// Set up a timer to send the message every 2 minute
ticker := time.NewTicker(2 * time.Minute)
// Use a goroutine to repeatedly send the message
go func() {
for range ticker.C {
sendTopCoinsMessage(bot)
}
}()
// Block the main goroutine to keep the program running
select {}
}
func sendTopCoinsMessage(bot *tgbotapi.BotAPI) {
urlEth := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=ethereum"
urlBtc := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=bitcoin"
urlSol := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=solana"
urlAda := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=cardano"
urlDot := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=polkadot"
urlXlm := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=stellar"
urlAtom := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=cosmos"
urlBnb := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=binancecoin"
priceBtc, erre := fetchPriceData(urlBtc)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceEth, erre := fetchPriceData(urlEth)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceSol, erre := fetchPriceData(urlSol)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceAda, erre := fetchPriceData(urlAda)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceDot, erre := fetchPriceData(urlDot)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceXlm, erre := fetchPriceData(urlXlm)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceAtom, erre := fetchPriceData(urlAtom)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceBnb, erre := fetchPriceData(urlBnb)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
// Prices with emojis and bold formatting
btcPrice := fmt.Sprintf("💰 *BTC*: $%.2f", priceBtc[0].CurrentPrice)
ethPrice := fmt.Sprintf("💰 *ETH*: $%.2f", priceEth[0].CurrentPrice)
solPrice := fmt.Sprintf("💰 *SOL*: $%.2f", priceSol[0].CurrentPrice)
adaPrice := fmt.Sprintf("💰 *ADA*: $%.2f", priceAda[0].CurrentPrice)
dotPrice := fmt.Sprintf("💰 *DOT*: $%.2f", priceDot[0].CurrentPrice)
xlmPrice := fmt.Sprintf("💰 *XLM*: $%.2f", priceXlm[0].CurrentPrice)
atomPrice := fmt.Sprintf("💰 *ATOM*: $%.2f", priceAtom[0].CurrentPrice)
bnbPrice := fmt.Sprintf("💰 *BNB*: $%.2f", priceBnb[0].CurrentPrice)
// Construct the message with Markdown formatting
messageFormat := fmt.Sprintf(`
*Top Coins Prices* 📈
%s
%s
%s
%s
%s
%s
%s
%s
🚀 Stay tuned for more updates! 🌕
`, btcPrice, ethPrice, solPrice, adaPrice, dotPrice, xlmPrice, atomPrice, bnbPrice)
msg := tgbotapi.NewMessageToChannel("@top_coins_price_alerts", messageFormat)
msg.ParseMode = "Markdown"
_, err := bot.Send(msg)
if err != nil {
log.Printf("Error sending message: %v", err)
}
}
Now paste following code in the prices.go file.
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type CoinData struct {
ID string `json:"id"`
Symbol string `json:"symbol"`
Name string `json:"name"`
CurrentPrice float64 `json:"current_price"`
MarketCap int64 `json:"market_cap"`
MarketCapRank int `json:"market_cap_rank"`
TotalVolume int64 `json:"total_volume"`
High24h float64 `json:"high_24h"`
Low24h float64 `json:"low_24h"`
PriceChange24h float64 `json:"price_change_24h"`
PriceChangePct24h float64 `json:"price_change_percentage_24h"`
CirculatingSupply float64 `json:"circulating_supply"`
TotalSupply float64 `json:"total_supply"`
MaxSupply *float64 `json:"max_supply"`
ATH float64 `json:"ath"`
ATHChangePct float64 `json:"ath_change_percentage"`
ATHDate string `json:"ath_date"`
ATL float64 `json:"atl"`
ATLChangePct float64 `json:"atl_change_percentage"`
ATLDate string `json:"atl_date"`
ROI struct {
Times float64 `json:"times"`
Currency string `json:"currency"`
Percentage float64 `json:"percentage"`
} `json:"roi"`
LastUpdated string `json:"last_updated"`
}
func fetchPriceData(url string) ([]CoinData, error) {
// Make a GET request to the API
resp, err := http.Get(url)
if err != nil {
return nil, err
}
defer resp.Body.Close()
// Check the response status code
if resp.StatusCode != http.StatusOK {
return nil, fmt.Errorf("API request failed with status code: %d", resp.StatusCode)
}
// Decode the JSON response into a slice of CoinData structs
var data []CoinData
err = json.NewDecoder(resp.Body).Decode(&data)
if err != nil {
return nil, err
}
return data, nil
Create the .env file
Create the .env file in the root of the project and initialize the BOT_TOKEN variable in the .env file.
Build & Run Project
Now build and run the project with the following command.
go build
go run main.go prices.go
The bot will start sending the coins price message every 2 minutes.
We have successfully built the crypto price bot telegram using Golang.
Conclusion
Congratulations, fellow coder! We navigated the creation of a Telegram bot crypto price alerts using Golang. With BotFather as your guide, you've equipped the power of Telegram channels to keep the crypto community informed and engaged. Armed with Golang, you've built a robust bot that delivers real-time updates on top cryptocurrencies.
This journey isn't just about prices and programming; it's about empowering yourself to explore endless possibilities for the best Telegram bot. Keep coding, keep innovating, and who knows what groundbreaking bot you'll create next? Cheers to your coding adventures and may your bots thrive in the vast digital landscape!
We trust this guide has refined your understanding of telegram bots. So, if you are craving more insights and expert advice? Explore our diverse range of tutorial blog posts or contact us. If you have questions or need to hire a proficient Golang developer, we are only a message away. Elevate your journey in the world of artificial intelligence today!
Table of Contents
Ever wondered how to create your very own cryptocurrency price alert Telegram bot using Golang? If so, we'll guide you through the process of building a bot that keeps you updated on the latest crypto prices, all while exploring the powerful combination of Telegram and Golang. Let's get on this coding adventure together!
So, cryptocurrency is a digital currency, which is another form of payment created using encryption algorithms used for trading, buying, and selling goods and other tokens, sometimes through crypto trading bots. However, one of the major uses of crypto through telegram bot Golang tutorial is to give awareness about the community of the crypto market crashes or cool offs. Likewise, telegram is a communication medium and one of its uses is to build bots that interact with users, groups, and channels. Here, we will learn to build a cryptocurrency alert telegram price bot using Golang.
Create a bot on Telegram
To create a bot on Telegram, we have to use BotFather which is the one of bot to rule all the bots. That is used to create new bot accounts and manage your existing bots. Now Open your telegram account and go to https://t.me/BotFather. Use the Forward slash / symbol that will give you the list of telegram bot commands.
Enter the /newbot command to create a new bot. It will ask you the name of your bot. Enter the username of the bot according to the requirements of BotFather
After that BotFather will give you the token to access the HTTP telegram bot API that we will use in our Golang program. Keep your token secure and store it safely, it can be used by anyone to control your bot.
Create a Telegram Bot Channel
We need an alert channel to send crypto price alerts by our telegram bot Golang where users get the alerts. To create a bot channel telegram, Open the Telegram app and click on the top right of the Chats bar on the Create a button that shows a popup to create a new channel.
Add Bot to Telegram Channel
Now add the created bot to the telegram channel. Open the Telegram Channel, go to channel info, click on the subscriber's button, and add the bot as a subscriber to the channel.
Now we implement bot logic in Golang that sends the crypto alert in the channel.
Install Golang
To use Golang, first install the Golang version must be 1.19 or higher. Go to the installation link: https://go.dev/doc/install. Select the OS of your local machine and download the top Golang framework and install it on your machine.
Verify the Go Version
To verify the Go installation version, enter the following command on the terminal.
go version
Create a Project
Create the Golang project to build the bot alert functionality. Now create a new folder with the name telegram-bot-golang. Open the folder in VS Code IDE and create new files main.go and prices.go in it.
Run the following commands:
go init telegram-bot-golang
go mod tidy
Paste the following code in the main.go file
package main
import (
"fmt"
"log"
"os"
"time"
tgbotapi "github.com/go-telegram-bot-api/telegram-bot-api/v5"
"github.com/joho/godotenv"
)
func main() {
err := godotenv.Load(".env")
if err != nil {
log.Fatalf("Error loading .env file")
}
bot, err := tgbotapi.NewBotAPI(os.Getenv("BOT_TOKEN"))
if err != nil {
log.Panic(err)
}
bot.Debug = true
log.Printf("Authorized on account %s", bot.Self.UserName)
sendTopCoinsMessage(bot)
// Set up a timer to send the message every 2 minute
ticker := time.NewTicker(2 * time.Minute)
// Use a goroutine to repeatedly send the message
go func() {
for range ticker.C {
sendTopCoinsMessage(bot)
}
}()
// Block the main goroutine to keep the program running
select {}
}
func sendTopCoinsMessage(bot *tgbotapi.BotAPI) {
urlEth := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=ethereum"
urlBtc := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=bitcoin"
urlSol := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=solana"
urlAda := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=cardano"
urlDot := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=polkadot"
urlXlm := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=stellar"
urlAtom := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=cosmos"
urlBnb := "https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd&ids=binancecoin"
priceBtc, erre := fetchPriceData(urlBtc)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceEth, erre := fetchPriceData(urlEth)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceSol, erre := fetchPriceData(urlSol)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceAda, erre := fetchPriceData(urlAda)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceDot, erre := fetchPriceData(urlDot)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceXlm, erre := fetchPriceData(urlXlm)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceAtom, erre := fetchPriceData(urlAtom)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
priceBnb, erre := fetchPriceData(urlBnb)
if erre != nil {
log.Printf("Error price fetching: %v", erre)
}
// Prices with emojis and bold formatting
btcPrice := fmt.Sprintf("💰 *BTC*: $%.2f", priceBtc[0].CurrentPrice)
ethPrice := fmt.Sprintf("💰 *ETH*: $%.2f", priceEth[0].CurrentPrice)
solPrice := fmt.Sprintf("💰 *SOL*: $%.2f", priceSol[0].CurrentPrice)
adaPrice := fmt.Sprintf("💰 *ADA*: $%.2f", priceAda[0].CurrentPrice)
dotPrice := fmt.Sprintf("💰 *DOT*: $%.2f", priceDot[0].CurrentPrice)
xlmPrice := fmt.Sprintf("💰 *XLM*: $%.2f", priceXlm[0].CurrentPrice)
atomPrice := fmt.Sprintf("💰 *ATOM*: $%.2f", priceAtom[0].CurrentPrice)
bnbPrice := fmt.Sprintf("💰 *BNB*: $%.2f", priceBnb[0].CurrentPrice)
// Construct the message with Markdown formatting
messageFormat := fmt.Sprintf(`
*Top Coins Prices* 📈
%s
%s
%s
%s
%s
%s
%s
%s
🚀 Stay tuned for more updates! 🌕
`, btcPrice, ethPrice, solPrice, adaPrice, dotPrice, xlmPrice, atomPrice, bnbPrice)
msg := tgbotapi.NewMessageToChannel("@top_coins_price_alerts", messageFormat)
msg.ParseMode = "Markdown"
_, err := bot.Send(msg)
if err != nil {
log.Printf("Error sending message: %v", err)
}
}
Now paste following code in the prices.go file.
package main
import (
"encoding/json"
"fmt"
"net/http"
)
type CoinData struct {
ID string `json:"id"`
Symbol string `json:"symbol"`
Name string `json:"name"`
CurrentPrice float64 `json:"current_price"`
MarketCap int64 `json:"market_cap"`
MarketCapRank int `json:"market_cap_rank"`
TotalVolume int64 `json:"total_volume"`
High24h float64 `json:"high_24h"`
Low24h float64 `json:"low_24h"`
PriceChange24h float64 `json:"price_change_24h"`
PriceChangePct24h float64 `json:"price_change_percentage_24h"`
CirculatingSupply float64 `json:"circulating_supply"`
TotalSupply float64 `json:"total_supply"`
MaxSupply *float64 `json:"max_supply"`
ATH float64 `json:"ath"`
ATHChangePct float64 `json:"ath_change_percentage"`
ATHDate string `json:"ath_date"`
ATL float64 `json:"atl"`
ATLChangePct float64 `json:"atl_change_percentage"`
ATLDate string `json:"atl_date"`
ROI struct {
Times float64 `json:"times"`
Currency string `json:"currency"`
Percentage float64 `json:"percentage"`
} `json:"roi"`
LastUpdated string `json:"last_updated"`
}
func fetchPriceData(url string) ([]CoinData, error) {
// Make a GET request to the API
resp, err := http.Get(url)
if err != nil {
return nil, err
}
defer resp.Body.Close()
// Check the response status code
if resp.StatusCode != http.StatusOK {
return nil, fmt.Errorf("API request failed with status code: %d", resp.StatusCode)
}
// Decode the JSON response into a slice of CoinData structs
var data []CoinData
err = json.NewDecoder(resp.Body).Decode(&data)
if err != nil {
return nil, err
}
return data, nil
Create the .env file
Create the .env file in the root of the project and initialize the BOT_TOKEN variable in the .env file.
Build & Run Project
Now build and run the project with the following command.
go build
go run main.go prices.go
The bot will start sending the coins price message every 2 minutes.
We have successfully built the crypto price bot telegram using Golang.
Conclusion
Congratulations, fellow coder! We navigated the creation of a Telegram bot crypto price alerts using Golang. With BotFather as your guide, you've equipped the power of Telegram channels to keep the crypto community informed and engaged. Armed with Golang, you've built a robust bot that delivers real-time updates on top cryptocurrencies.
This journey isn't just about prices and programming; it's about empowering yourself to explore endless possibilities for the best Telegram bot. Keep coding, keep innovating, and who knows what groundbreaking bot you'll create next? Cheers to your coding adventures and may your bots thrive in the vast digital landscape!
We trust this guide has refined your understanding of telegram bots. So, if you are craving more insights and expert advice? Explore our diverse range of tutorial blog posts or contact us. If you have questions or need to hire a proficient Golang developer, we are only a message away. Elevate your journey in the world of artificial intelligence today!
FAQS
Here's a general guide on how you can create a simple crypto Telegram bot using Python and a popular cryptocurrency API
Step 1
Chat with BotFather on Telegram to create a new bot and get the API token.
Step 2
Ensure Python is installed on your computer.
Step 3
Run pip install python-telegram-bot requests in the terminal.
Step 4
Use a code editor to create a script (e.g., crypto_bot.py).
Step 5
Paste the provided code, replacing 'YOUR_TELEGRAM_BOT_TOKEN'.
Step 6
Execute the script in the terminal.
Step 7
Start a chat with your bot on Telegram.
Use /start to begin and /price <symbol> for cryptocurrency prices.
To install Go (Golang) on windows, you can follow these general steps.
Download Go
Visit the official Go website.
Download the MSI installer for Windows.
Run Installer
Run the downloaded MSI installer.
Follow the installation prompts.
Verify Installation
Open a new command prompt or PowerShell.
Type the following command and press Enter:
bash
Copy code
go version
If installed correctly, you should see the Go version number. That's it! Go is now installed on your Windows system.
To integrate the Telegram library in Go, you can use the github.com/go-telegram-bot-api/telegram-bot-api package. Below are the steps to set up a basic Telegram bot in Go:
Install: Use go get command.
Create Bot: Obtain API token from BotFather on Telegram.
Write Go Code: Use the Telegram library for Go.
Run: Execute your Go program.
Test: Start a chat with your bot on Telegram and send a message.
Advanced: Refer to Telegram library documentation.