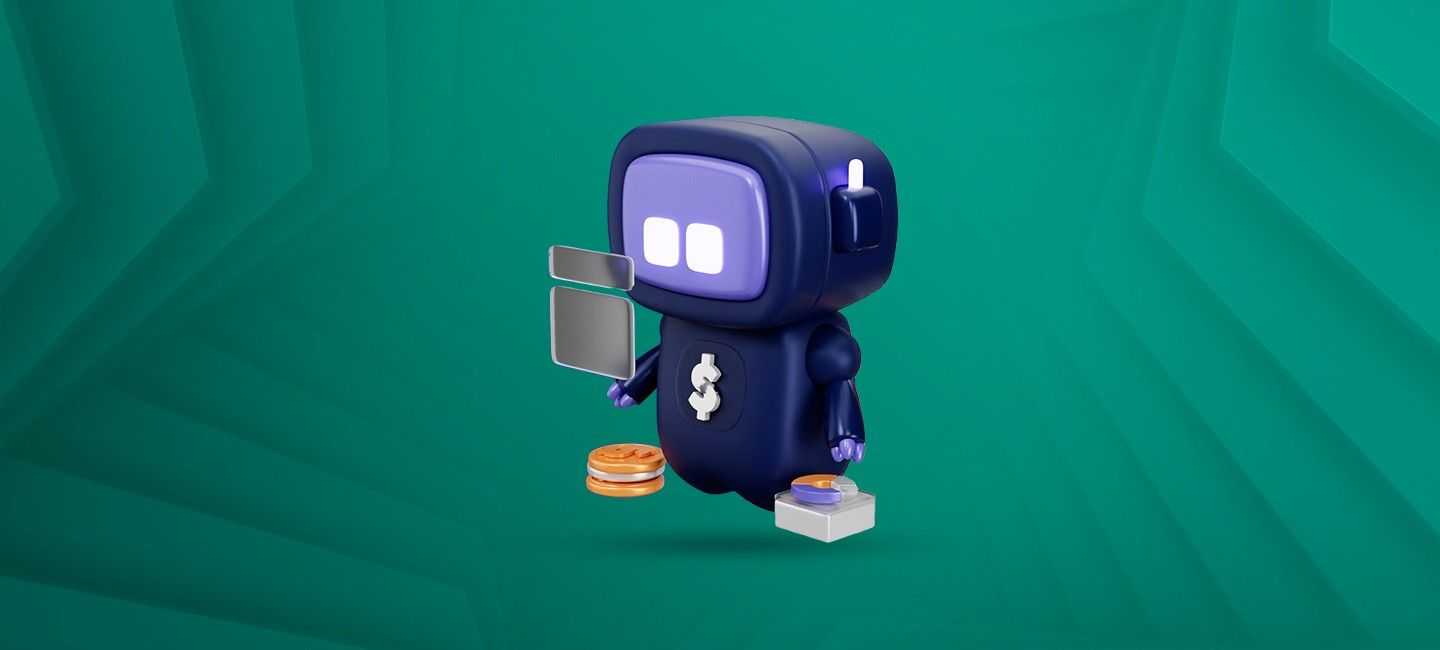
Crypto Trading Bot Development | A Complete Guide
Table of Contents
There are several factors and variables that trading depends on, which makes it complex even for experienced traders. If you want to build a crypto portfolio, but are looking for a quick and easy way to do so, you must be out looking for crypto bots. While there’s hundreds of companies offering different crypto trading bots, to get started you should consider making your own. If you are just starting your crypto bot would need only be simple.
Good news is, even a basic crypto trading bot is going to help you out. Another good news is, you would not have to pay fees to some company. More good news; A crypto bot can be made in three simple steps.
What is a Crypto Trading Bot?
Put simply, a Crypto Trading Bot is like having a digital assistant that trades cryptocurrencies for you. It's a computer program that never sleeps, always on the lookout for profitable opportunities in the market. By analyzing real-time data and using strategies you set, the bot makes smart decisions on when to buy and sell, aiming to boost your profits and reduce potential losses.
What is the Role of Crypto Trading Bots?
The key to trading is reducing the risk attached to a trade. The complex nature of trading, and the growing saturation of the trading world, requires a method of trading that is as risk free as possible, or at the very least, has a calculated risk. By taking out the sentimental factor, also known as human risk factor, and by leveraging bots' ability to compute and make decisions much quicker, the best crypto trading bot can prove to be profitable.
Because there are a number of factors involved in trading, there are several types of trading bots. In this article, we will build a Python Crypto Trading Bot.
What is a Technical Crypto Trading Bot?
Most of the trading bots nowadays are technical trading bots. These types of trading bots utilize mathematically calculated trading indicators, and predict the future prices, and make decisions to trade based on that to generate profit.
Prerequisites
Before we move on to the trading bot, there are a few decisions we need to make. The first is, deciding which cryptocurrency we are going to trade in. You can decide this by checking out conversion rates, and popularity, alongside latest trends. Pick one that is best suited for you. In this article we will be trading ETH and USDT.
The second is, what exchange are we going to trade on? For crypto trading, the options are countless, but the major decision is to choose between a Centralized Exchange, and a Decentralized Exchange.
Usually, the decision can be made by considering the following:
- How much fee am I willing to pay?
- Do I want a custodial or a non custodial wallet?
- Which crypto currencies do I want to trade in? Are all of them listed on the exchange?
In our trading bot, we will be using Binance, which is a Centralised Exchange, and as a low fee.
Lastly, and most importantly, have capital. To begin trading, you would need sufficient capital to start trading, pay fees, and incur a loss in the worst case scenario.
Once you have come to a consensus on these, we can start building the trading bot. As mentioned before, we will be doing so in three steps.
How to Make a Crypto Trading Bot in Python Just in Four Steps
Follow these four steps to create your own crypto trading bot in Python:
Step 1: Connect to Exchange
The first step is to connect to an exchange. For that, you need to have an account on the exchange, and an API key. In our bot, we will for now use the test binance network. Just by changing the network and API key, the main network can be used.
To create an account and API key you can use this. Once you have the account and the API key, you can start coding the trading bot.
from binance.client import Client
#init
api_key = #YOUR_API_KEY
api_secret = #YOUR_API_SECRET
client = Client(api_key, api_secret)
client.API_URL = 'https://testnet.binance.vision/api' #test url
cryptoSymbol = 'ETHUSDT'#decided pair
Using the python Binance library, we can connect to the exchange in just a few lines. In the code, replace the comments with your API key and your API secret, and then when you run the code, the connection would be made to the binance test network.
Step 2: Get Live Data
Next step is to get the live data for ETH USDT from the exchange. This is the data on which we will be calculating the technical indicators for crypto bot trading strategies. The data we require from the exchange is historical data or data in the form of candle sticks. This data gives us the date, the opening price, the closing price, and the high and low of the cryptocurrency. We will store this data in a dataframe for an organized storage and access using keys.
import pandas as pd
def fetchData():
#setting the interval for getting historical data
interval = '5m'
#get timestamp of the earliest timestamp data available
timestamp = client._get_earliest_valid_timestamp(cryptoSymbol, interval)
#get candle stick data
historialCandles = client.get_historical_klines(cryptoSymbol, interval, timestamp, limit=1000)
#place required data in datafram
for line in historialCandles:
del line[5:]
dataFrame = pd.DataFrame(historialCandles, columns=['date', 'open', 'high', 'low', 'close'])
return dataFrame
Here, we are getting the data over a time interval of 5 minutes. Then we return the data that we got from the Finance API.
Step 3: Trading Algorithm Application
For our trading, our algorithm would use two technical indicators. First is Moving Average Convergence/Divergence, and second is Relative Strength Index. To understand these two mathematical concepts, I’d suggest reading up about them a little.
Our trading strategy would be the following:
If MACD gives the buy signal, and RSI is in the oversold region, BUY. If MACD gives the sell signal, and RSI is in the overbought region, SELL. Else, wait for one of the signals.
To start, let’s first see our MACD implementation.
D'] = macdDF['Moving Average Fast'] - macdDF['Moving Average Slow']
#signal
macdDF['Signal'] = macdDF['MACD'].ewm(span=signal, min_periods= signal).mean()
macdDF['Hist'] = macdDF['MACD'] - macdDF['Signal']
return macdDFdef calculateMACD(dataFrame, fast, slow, signal):
macdDF = pd.DataFrame()
#calculate exponential moving average
macdDF['Moving Average Fast'] = dataFrame['close'].ewm(span=fast, min_periods=fast).mean()
macdDF['Moving Average Slow'] = dataFrame['close'].ewm(span=slow, min_periods=slow).mean()
#macd
macdDF['MAC
Then, RSI implementation.
def calculateRSI(dataFrame):
rsiDF = pd.DataFrame()
#Calculate Diff
rsiDF['diff'] = dataFrame['price'].diff(1)
#Calculate Gain and Loss
rsiDF['gain'] = rsiDF['diff'].clip(lower=0).round(2)
rsiDF['loss'] = rsiDF['diff'].clip(upper=0).abs().round(2)
#Calculate Avg Gain and Loss
timePeriod = 14
rsiDF['avgGain'] = rsiDF['gain'].rolling(window=timePeriod, min_periods=timePeriod).mean()[:timePeriod+1]
rsiDF['avgLoss'] = rsiDF['loss'].rolling(window=timePeriod, min_periods=timePeriod).mean()[:timePeriod+1]
#Get WSM avg
for i, row in enumerate(rsiDF['avgGain'].iloc[timePeriod+1:]):
rsiDF['avgGain'].iloc[i + timePeriod + 1] =\
(rsiDF['avgGain'].iloc[i + timePeriod] *
(timePeriod - 1) +
rsiDF['gain'].iloc[i + timePeriod + 1])\
/ timePeriod
for i, row in enumerate(rsiDF['avgLoss'].iloc[timePeriod+1:]):
rsiDF['avgLoss'].iloc[i + timePeriod + 1] =\
(rsiDF['avgLoss'].iloc[i + timePeriod] *
(timePeriod - 1) +
rsiDF['loss'].iloc[i + timePeriod + 1])\
/ timePeriod
#calculate RS value
rsiDF['rs'] = rsiDF['avgGain'] / rsiDF['avgLoss']
#calculate RSI
rsiDF['rsi'] = 100 - (100 / (1.0 + rsiDF['rs']))
return rsiDF
Then, we use these to check if we should buy or sell.
import numpy as np
def computeTechnicalIndicators(dataFrame):
macdStatus, rsiStatus = 'WAIT', 'WAIT'
#Set index of the dataframe to date
dataFrame.set_index('date', inplace=True)
#Change unit to milliseconds
dataFrame.index = pd.to_datetime(dataFrame.index, unit='ms')
macdDF = calculateMACD(dataFrame, 12, 26, 9)
lastHist = macdDF['Hist'].iloc[-1]
prevHist = macdDF['Hist'].iloc[-2]
if not np.isnan(prevHist) and not np.isnan(lastHist):
crossover = (abs(lastHist+prevHist)) != (abs(lastHist)) + (abs(prevHist))
if crossover:
macdStatus = 'BUY' if lastHist > 0 else 'SELL'
print(macdStatus)
if macdStatus != 'WAIT':
rsi = calculateRSI(dataFrame)
lastRsi = rsi['rsi'].iloc[-1]
print(rsi)
if(lastRsi <= 30):
rsiStatus = 'BUY'
elif (lastRsi >= 70):
rsiStatus = 'SELL'
else:
print("MACD Calculations suggest to WAIT")
return rsiStatus
Step 4: Run trade
Once we have gotten a decision based on our trading algorithm, we execute the trade. Again, we use Binance APIs for that.
import time
from binance.enums import *
from binance.exceptions import BinanceAPIException, BinanceOrderException
def executeTrade():
while(1):
dataFrame = fetchData()
rsi = computeTechnicalIndicators(dataFrame)
currentlyHolding = False
if rsi == 'BUY' and not currentlyHolding:
print("Placing BUY order")
currentlyHolding = True
try:
buy_limit = client.order_market_buy(symbol=cryptoSymbol, quantity=100, price=2000)
except BinanceAPIException as e:
# error handling goes here
print(e)
except BinanceOrderException as e:
# error handling goes here
print(e)
elif rsi == 'SELL' and currentlyHolding:
print("Placing SELL order")
try:
market_order = client.order_market_sell(symbol=cryptoSymbol, quantity=100)
except BinanceAPIException as e:
# error handling goes here
print(e)
except BinanceOrderException as e:
# error handling goes here
print(e)
currentlyHolding=False
time.sleep(60*5) #interval is 5 minutes
return
executeTrade()
That concludes our basic trading bot in three steps. The auto crypto trading bot gets new data every five minutes, runs the trading algorithm, and buys, sells, or waits based on these.
Conclusion
Writing your own open source crypto trading bots for your own specific case is straight forward. This bot can be improved as well, as this is a simple trading bot. Some ways to improve it are:
- Adding more technical indicators
- Adding more states to the bot
You can check out the complete code on Github here. If you still want a more customized trading bot, and seek consultation from experienced traders and experts on trading bot development, we at InvoBlox would love to help out.
Table of Contents
There are several factors and variables that trading depends on, which makes it complex even for experienced traders. If you want to build a crypto portfolio, but are looking for a quick and easy way to do so, you must be out looking for crypto bots. While there’s hundreds of companies offering different crypto trading bots, to get started you should consider making your own. If you are just starting your crypto bot would need only be simple.
Good news is, even a basic crypto trading bot is going to help you out. Another good news is, you would not have to pay fees to some company. More good news; A crypto bot can be made in three simple steps.
What is a Crypto Trading Bot?
Put simply, a Crypto Trading Bot is like having a digital assistant that trades cryptocurrencies for you. It's a computer program that never sleeps, always on the lookout for profitable opportunities in the market. By analyzing real-time data and using strategies you set, the bot makes smart decisions on when to buy and sell, aiming to boost your profits and reduce potential losses.
What is the Role of Crypto Trading Bots?
The key to trading is reducing the risk attached to a trade. The complex nature of trading, and the growing saturation of the trading world, requires a method of trading that is as risk free as possible, or at the very least, has a calculated risk. By taking out the sentimental factor, also known as human risk factor, and by leveraging bots' ability to compute and make decisions much quicker, the best crypto trading bot can prove to be profitable.
Because there are a number of factors involved in trading, there are several types of trading bots. In this article, we will build a Python Crypto Trading Bot.
What is a Technical Crypto Trading Bot?
Most of the trading bots nowadays are technical trading bots. These types of trading bots utilize mathematically calculated trading indicators, and predict the future prices, and make decisions to trade based on that to generate profit.
Prerequisites
Before we move on to the trading bot, there are a few decisions we need to make. The first is, deciding which cryptocurrency we are going to trade in. You can decide this by checking out conversion rates, and popularity, alongside latest trends. Pick one that is best suited for you. In this article we will be trading ETH and USDT.
The second is, what exchange are we going to trade on? For crypto trading, the options are countless, but the major decision is to choose between a Centralized Exchange, and a Decentralized Exchange.
Usually, the decision can be made by considering the following:
- How much fee am I willing to pay?
- Do I want a custodial or a non custodial wallet?
- Which crypto currencies do I want to trade in? Are all of them listed on the exchange?
In our trading bot, we will be using Binance, which is a Centralised Exchange, and as a low fee.
Lastly, and most importantly, have capital. To begin trading, you would need sufficient capital to start trading, pay fees, and incur a loss in the worst case scenario.
Once you have come to a consensus on these, we can start building the trading bot. As mentioned before, we will be doing so in three steps.
How to Make a Crypto Trading Bot in Python Just in Four Steps
Follow these four steps to create your own crypto trading bot in Python:
Step 1: Connect to Exchange
The first step is to connect to an exchange. For that, you need to have an account on the exchange, and an API key. In our bot, we will for now use the test binance network. Just by changing the network and API key, the main network can be used.
To create an account and API key you can use this. Once you have the account and the API key, you can start coding the trading bot.
from binance.client import Client
#init
api_key = #YOUR_API_KEY
api_secret = #YOUR_API_SECRET
client = Client(api_key, api_secret)
client.API_URL = 'https://testnet.binance.vision/api' #test url
cryptoSymbol = 'ETHUSDT'#decided pair
Using the python Binance library, we can connect to the exchange in just a few lines. In the code, replace the comments with your API key and your API secret, and then when you run the code, the connection would be made to the binance test network.
Step 2: Get Live Data
Next step is to get the live data for ETH USDT from the exchange. This is the data on which we will be calculating the technical indicators for crypto bot trading strategies. The data we require from the exchange is historical data or data in the form of candle sticks. This data gives us the date, the opening price, the closing price, and the high and low of the cryptocurrency. We will store this data in a dataframe for an organized storage and access using keys.
import pandas as pd
def fetchData():
#setting the interval for getting historical data
interval = '5m'
#get timestamp of the earliest timestamp data available
timestamp = client._get_earliest_valid_timestamp(cryptoSymbol, interval)
#get candle stick data
historialCandles = client.get_historical_klines(cryptoSymbol, interval, timestamp, limit=1000)
#place required data in datafram
for line in historialCandles:
del line[5:]
dataFrame = pd.DataFrame(historialCandles, columns=['date', 'open', 'high', 'low', 'close'])
return dataFrame
Here, we are getting the data over a time interval of 5 minutes. Then we return the data that we got from the Finance API.
Step 3: Trading Algorithm Application
For our trading, our algorithm would use two technical indicators. First is Moving Average Convergence/Divergence, and second is Relative Strength Index. To understand these two mathematical concepts, I’d suggest reading up about them a little.
Our trading strategy would be the following:
If MACD gives the buy signal, and RSI is in the oversold region, BUY. If MACD gives the sell signal, and RSI is in the overbought region, SELL. Else, wait for one of the signals.
To start, let’s first see our MACD implementation.
D'] = macdDF['Moving Average Fast'] - macdDF['Moving Average Slow']
#signal
macdDF['Signal'] = macdDF['MACD'].ewm(span=signal, min_periods= signal).mean()
macdDF['Hist'] = macdDF['MACD'] - macdDF['Signal']
return macdDFdef calculateMACD(dataFrame, fast, slow, signal):
macdDF = pd.DataFrame()
#calculate exponential moving average
macdDF['Moving Average Fast'] = dataFrame['close'].ewm(span=fast, min_periods=fast).mean()
macdDF['Moving Average Slow'] = dataFrame['close'].ewm(span=slow, min_periods=slow).mean()
#macd
macdDF['MAC
Then, RSI implementation.
def calculateRSI(dataFrame):
rsiDF = pd.DataFrame()
#Calculate Diff
rsiDF['diff'] = dataFrame['price'].diff(1)
#Calculate Gain and Loss
rsiDF['gain'] = rsiDF['diff'].clip(lower=0).round(2)
rsiDF['loss'] = rsiDF['diff'].clip(upper=0).abs().round(2)
#Calculate Avg Gain and Loss
timePeriod = 14
rsiDF['avgGain'] = rsiDF['gain'].rolling(window=timePeriod, min_periods=timePeriod).mean()[:timePeriod+1]
rsiDF['avgLoss'] = rsiDF['loss'].rolling(window=timePeriod, min_periods=timePeriod).mean()[:timePeriod+1]
#Get WSM avg
for i, row in enumerate(rsiDF['avgGain'].iloc[timePeriod+1:]):
rsiDF['avgGain'].iloc[i + timePeriod + 1] =\
(rsiDF['avgGain'].iloc[i + timePeriod] *
(timePeriod - 1) +
rsiDF['gain'].iloc[i + timePeriod + 1])\
/ timePeriod
for i, row in enumerate(rsiDF['avgLoss'].iloc[timePeriod+1:]):
rsiDF['avgLoss'].iloc[i + timePeriod + 1] =\
(rsiDF['avgLoss'].iloc[i + timePeriod] *
(timePeriod - 1) +
rsiDF['loss'].iloc[i + timePeriod + 1])\
/ timePeriod
#calculate RS value
rsiDF['rs'] = rsiDF['avgGain'] / rsiDF['avgLoss']
#calculate RSI
rsiDF['rsi'] = 100 - (100 / (1.0 + rsiDF['rs']))
return rsiDF
Then, we use these to check if we should buy or sell.
import numpy as np
def computeTechnicalIndicators(dataFrame):
macdStatus, rsiStatus = 'WAIT', 'WAIT'
#Set index of the dataframe to date
dataFrame.set_index('date', inplace=True)
#Change unit to milliseconds
dataFrame.index = pd.to_datetime(dataFrame.index, unit='ms')
macdDF = calculateMACD(dataFrame, 12, 26, 9)
lastHist = macdDF['Hist'].iloc[-1]
prevHist = macdDF['Hist'].iloc[-2]
if not np.isnan(prevHist) and not np.isnan(lastHist):
crossover = (abs(lastHist+prevHist)) != (abs(lastHist)) + (abs(prevHist))
if crossover:
macdStatus = 'BUY' if lastHist > 0 else 'SELL'
print(macdStatus)
if macdStatus != 'WAIT':
rsi = calculateRSI(dataFrame)
lastRsi = rsi['rsi'].iloc[-1]
print(rsi)
if(lastRsi <= 30):
rsiStatus = 'BUY'
elif (lastRsi >= 70):
rsiStatus = 'SELL'
else:
print("MACD Calculations suggest to WAIT")
return rsiStatus
Step 4: Run trade
Once we have gotten a decision based on our trading algorithm, we execute the trade. Again, we use Binance APIs for that.
import time
from binance.enums import *
from binance.exceptions import BinanceAPIException, BinanceOrderException
def executeTrade():
while(1):
dataFrame = fetchData()
rsi = computeTechnicalIndicators(dataFrame)
currentlyHolding = False
if rsi == 'BUY' and not currentlyHolding:
print("Placing BUY order")
currentlyHolding = True
try:
buy_limit = client.order_market_buy(symbol=cryptoSymbol, quantity=100, price=2000)
except BinanceAPIException as e:
# error handling goes here
print(e)
except BinanceOrderException as e:
# error handling goes here
print(e)
elif rsi == 'SELL' and currentlyHolding:
print("Placing SELL order")
try:
market_order = client.order_market_sell(symbol=cryptoSymbol, quantity=100)
except BinanceAPIException as e:
# error handling goes here
print(e)
except BinanceOrderException as e:
# error handling goes here
print(e)
currentlyHolding=False
time.sleep(60*5) #interval is 5 minutes
return
executeTrade()
That concludes our basic trading bot in three steps. The auto crypto trading bot gets new data every five minutes, runs the trading algorithm, and buys, sells, or waits based on these.
Conclusion
Writing your own open source crypto trading bots for your own specific case is straight forward. This bot can be improved as well, as this is a simple trading bot. Some ways to improve it are:
- Adding more technical indicators
- Adding more states to the bot
You can check out the complete code on Github here. If you still want a more customized trading bot, and seek consultation from experienced traders and experts on trading bot development, we at InvoBlox would love to help out.
FAQS
3Commas stands out as a favorite among cryptocurrency traders due to its user-friendly interface, allowing both beginners and seasoned traders to navigate the platform effortlessly.
The profitability of crypto trading bots is uncertain, much like the volatile cryptocurrency market they navigate. While some users report profits, there are no guarantees due to market fluctuations and other factors. A well-planned strategy and understanding the bot are crucial. Caution is advised, and only invest what you can afford to lose. It's a learning experience, and realistic expectations are essential.
To create a trading bot for Binance, get an API key, integrate Binance's API, code your strategy, backtest, paper trade, deploy, and monitor cautiously.